Answer:
The this pointer in C++ is a constant pointer that stores address of an object of a class. The type of this pointer is the type of object it is pointing to. For example, if we have a class named ‘sample’, the type of this pointer is ‘sample* const this’. (What is type of integer pointer ( int* ptr)? Its integer type as it points to int value. Similarly, type of ‘this’ pointer is object type it points to.)
Whenever we call a non-static member function of a class on some objects, a ‘this’ pointer get created and address of that object get stored in it and passed as a hidden argument to that function and in function body we can use this pointer as a local variable.
Function(myclass* const this’, arg1,arg2,…);
Example:
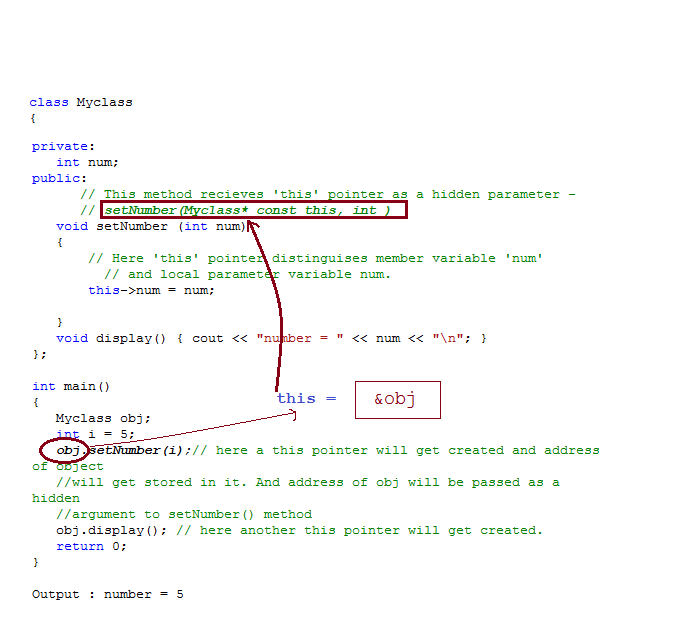
NOTES:
Important points
- this pointer is not available to static method of a class as calling static method doesn’t require object but class name only(however we can call static method using object also). For example Myclass:: myStaticMethod(int num); To test if this pointer is available to static method, write this method to above class Myclass. Compiler will flash an error “static member functions do not have ‘this’ pointers”.
static void myStaticFun(int num){ this->num = num; )
- Friend function doesn’t have this pointer.
- this pointer is rvalue not lvalue, so, can’t access address of it.
- In class constructor, also this pointer is available. When we create the object, compiler allocate memory for it and on constructor invocation, “this” pointer get passed internally. After that objects initialization is done.
To focus: sub questions
Q-when does this pointer in C++ get created?
Answer: when a non-static member function of a class gets called.
Q- what is a type of this pointer in C++?
Answer : The type of this pointer is the type of object it is pointing to.